学习笔记:使用 Cocos Creator 3.4.0 开发空战游戏 02——玩家飞机
开发工具
- Cocos Dashboard 1.0.20
- Cocos Creator 3.4.0
- Visual Studio Code 1.63
- Microsoft Edge 97.0.1072.69
创建玩家飞机
- 在
层级管理器
中 创建一个空节点, 命名为playerPlane
, 在资源管理器
中拖动的FBX
模型assets/res/model/plane01/plane01
到playerPlane
节点中, 并将节点playerPlane
下的模型名称plane01
(模型根节点) 改名为body
。
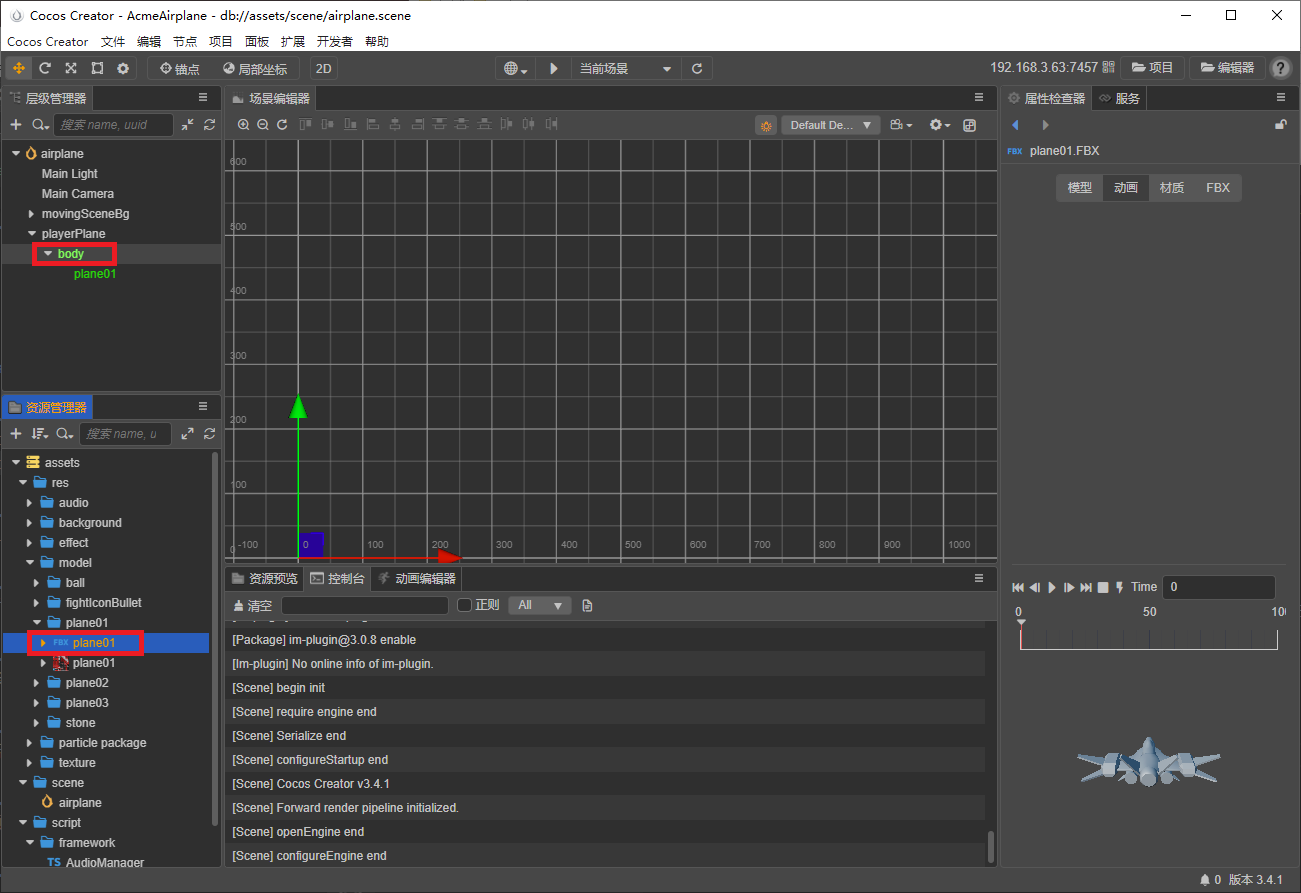
- 在
资源管理器
中点击选中assets/res/model/plane01
文件夹,右键
->创建
->材质
, 命名为playerPlane
。
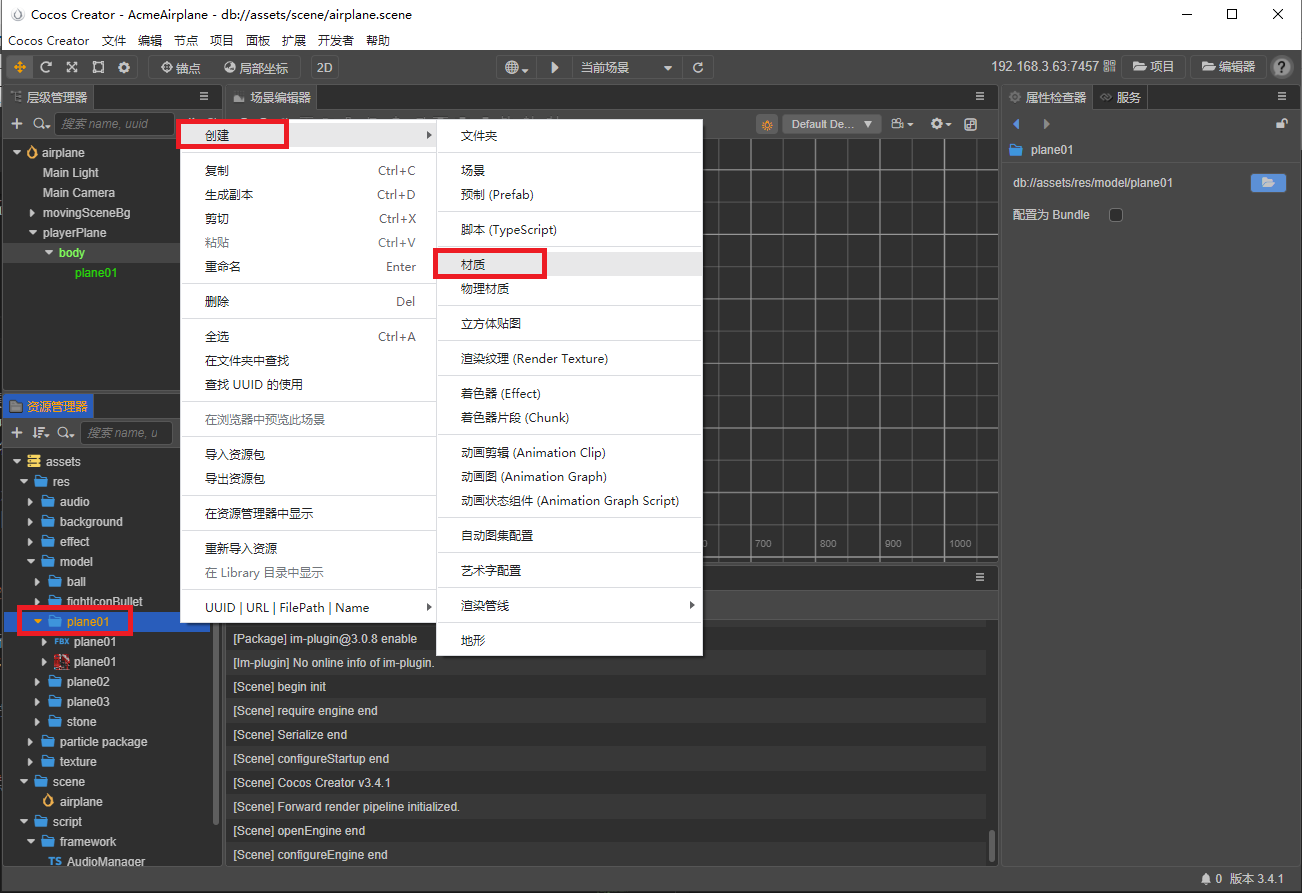
- 将材质
playerPlane
的属性Effect
更改为builtin-unlit
; 属性USE TEXTURE
打勾, 飞机图片plane01
拖动到MainTexture
, 保存材质。
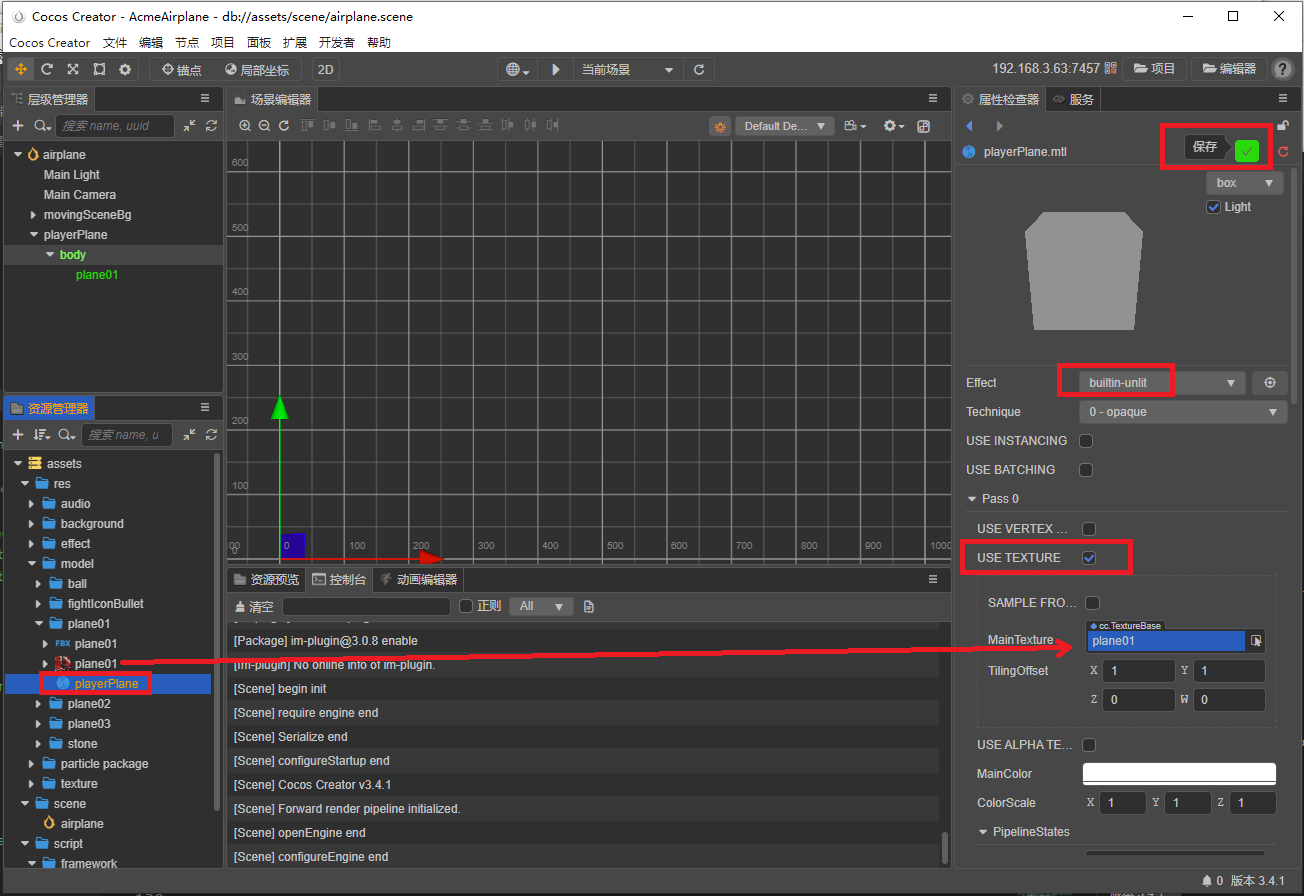
- 在
层级管理器
中, 点击选中playerPlane/body/plane01
节点, 将上一步创建的材质playerPlane
拖动到其属性检查器
中的Materials
[0], 保存场景。
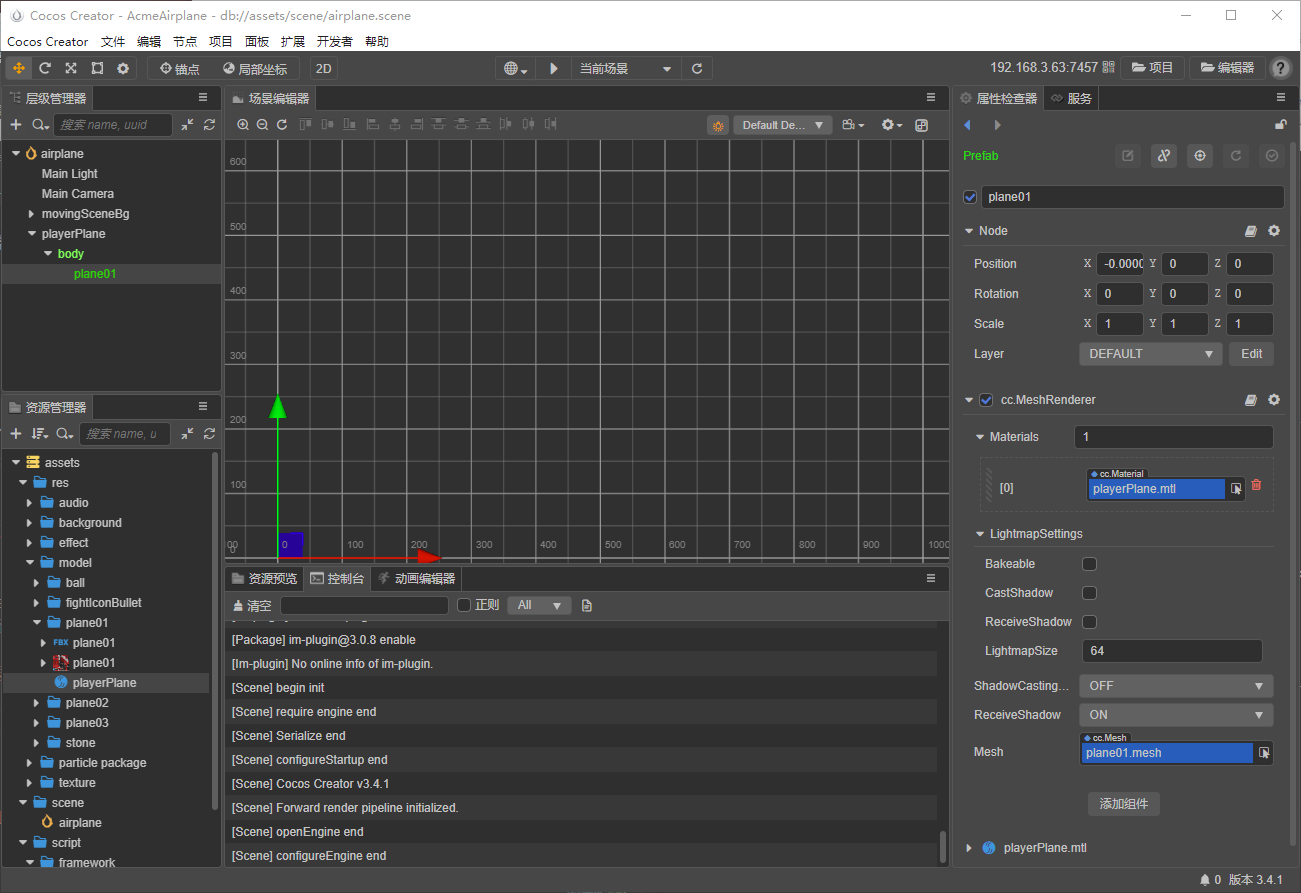
在
层级管理器
中点击选中playerPlane/body
节点, 修改缩放属性Scale
的值为:X:8, Y:8, Z:8
(放大8倍)。此时飞机与背景板重叠,在
层级管理器
中点击选中movingSceneBg
, 将其Position
的Y轴
修改为:-20
(背景往下移)。
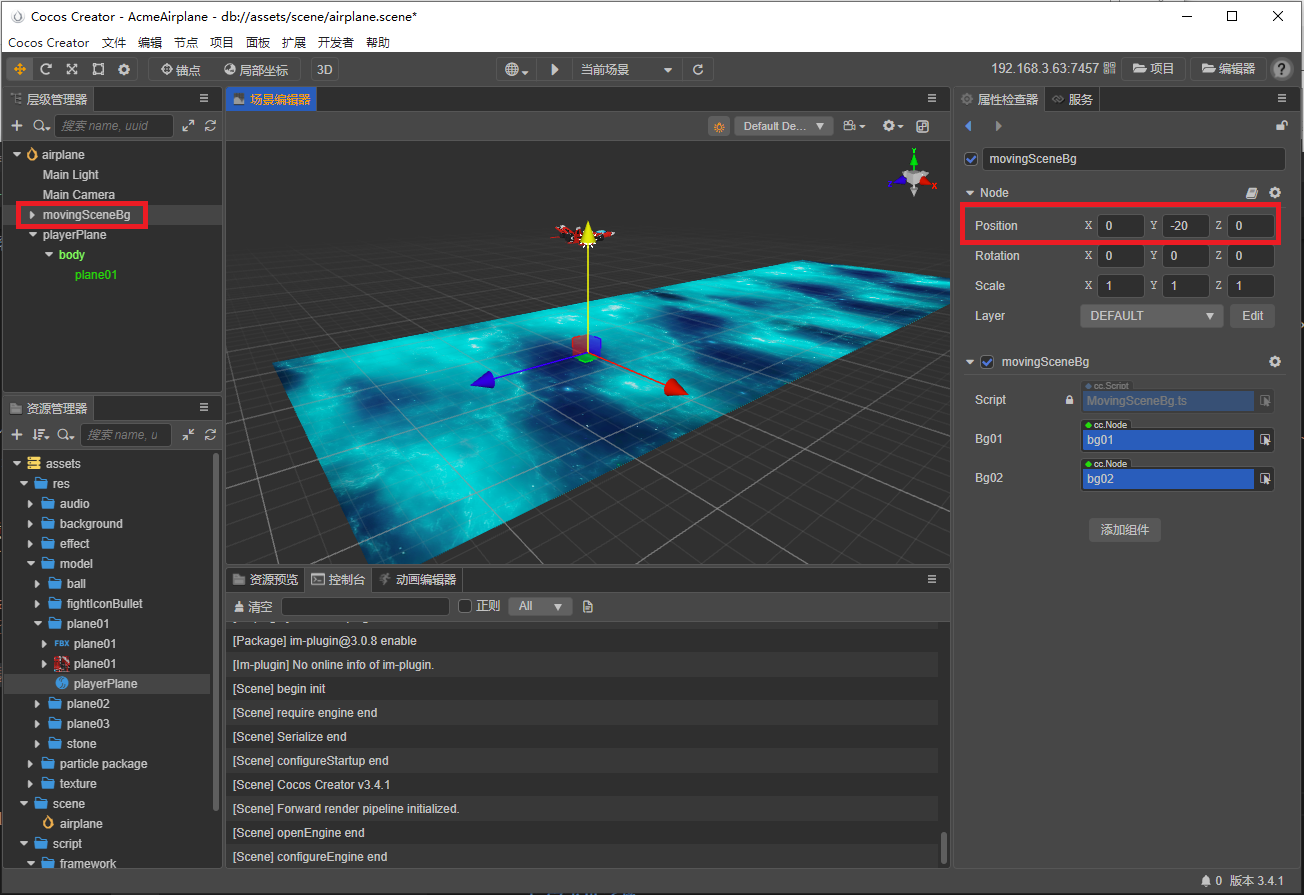
- 在
层级管理器
中点击选中playerPlane
节点,将其拖动到资源管理器
中的assets/res/model/plane01
文件夹制作
成预制
, 保存场景。
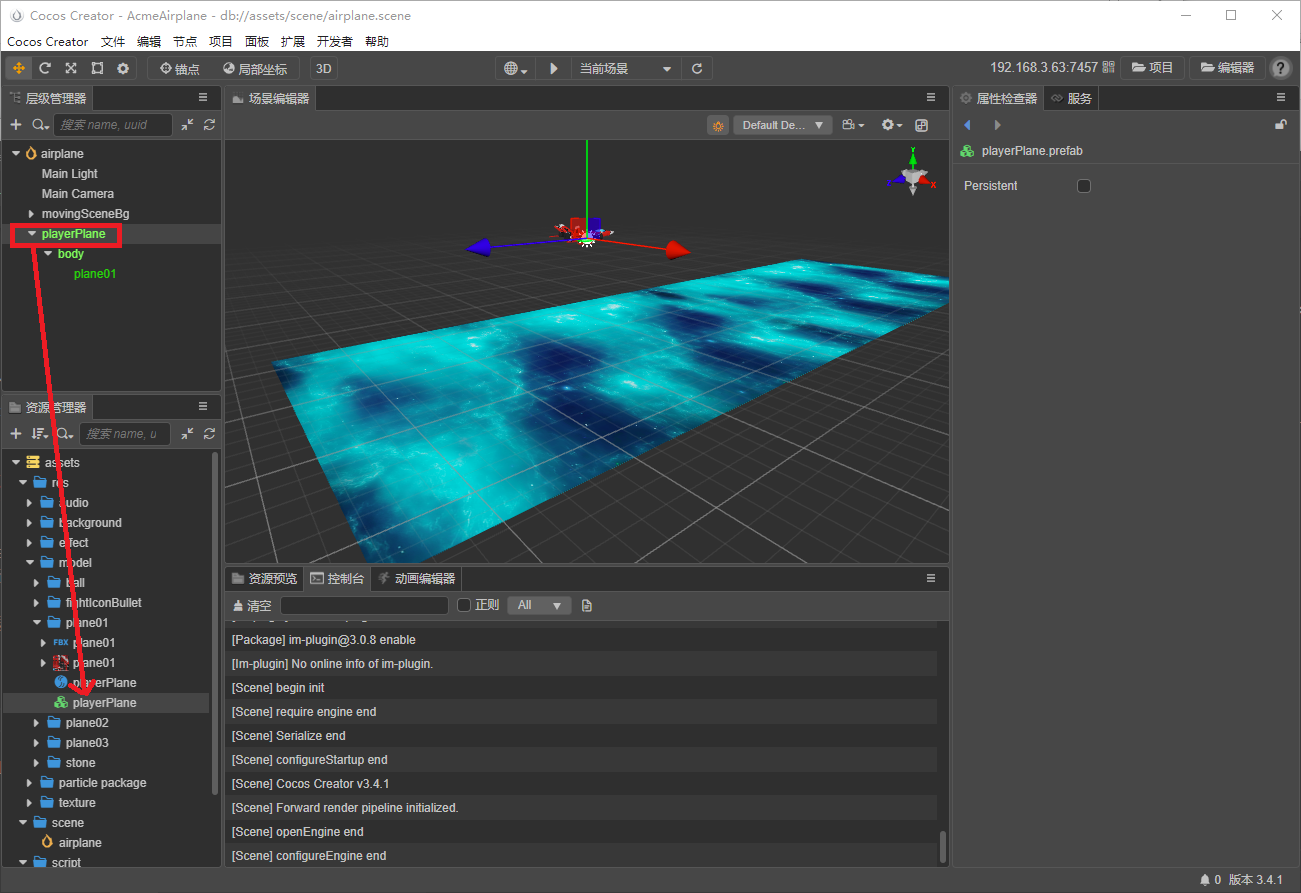
控制飞机移动
- 在
资源管理器
点击选中assets/script/
文件夹,右键
->创建
->文件夹
, 命名为ui
, 在该文件夹中添加脚本UIManager
, 双击打开该脚本进行编辑。
1 |
|
在
层级管理器
中点击选中场景airplane
,右键
->创建
->UI组件
->Canvas(画布)
, 名字默认。在
层级管理器
中点击选中Canvas
节点, 在属性检查器
点添加组件
->自定义脚本
->UIManager
, 将上一步编辑好的脚本UIManager
挂载到Canvas
根节点。将
层级管理器
中的playerPlane
节点拖到Canvas
节点属性检查器
的playerPlane
属性中, 修改playerPlaneSpeed
值为 8,保存场景,预览。
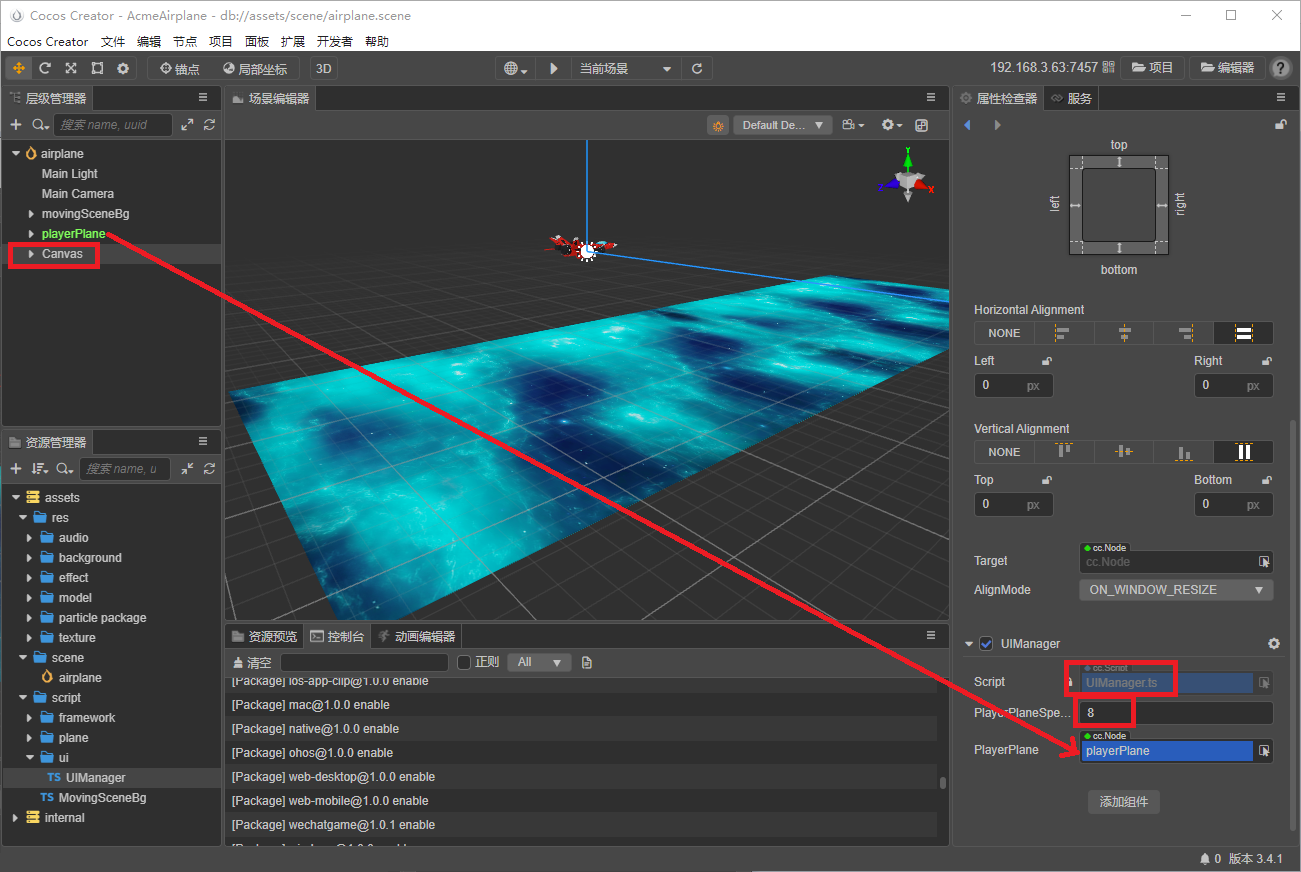
玩家飞机子弹
- 在
资源管理器
点击选中assets/res/effect/bullet/
文件夹,右键
->创建
->材质
, 命名为bullet01
, 修改Effect
为bulitin-unlit
, 此处子弹模型包含透明外环效果, 将Technique
修改为3-alpha-blend
模式; 选中USE TEXTURE
, 将图片bullet01
拖动到属性检查器
中的属性MainTexture
, 在属性检查器
右上角点保存
。
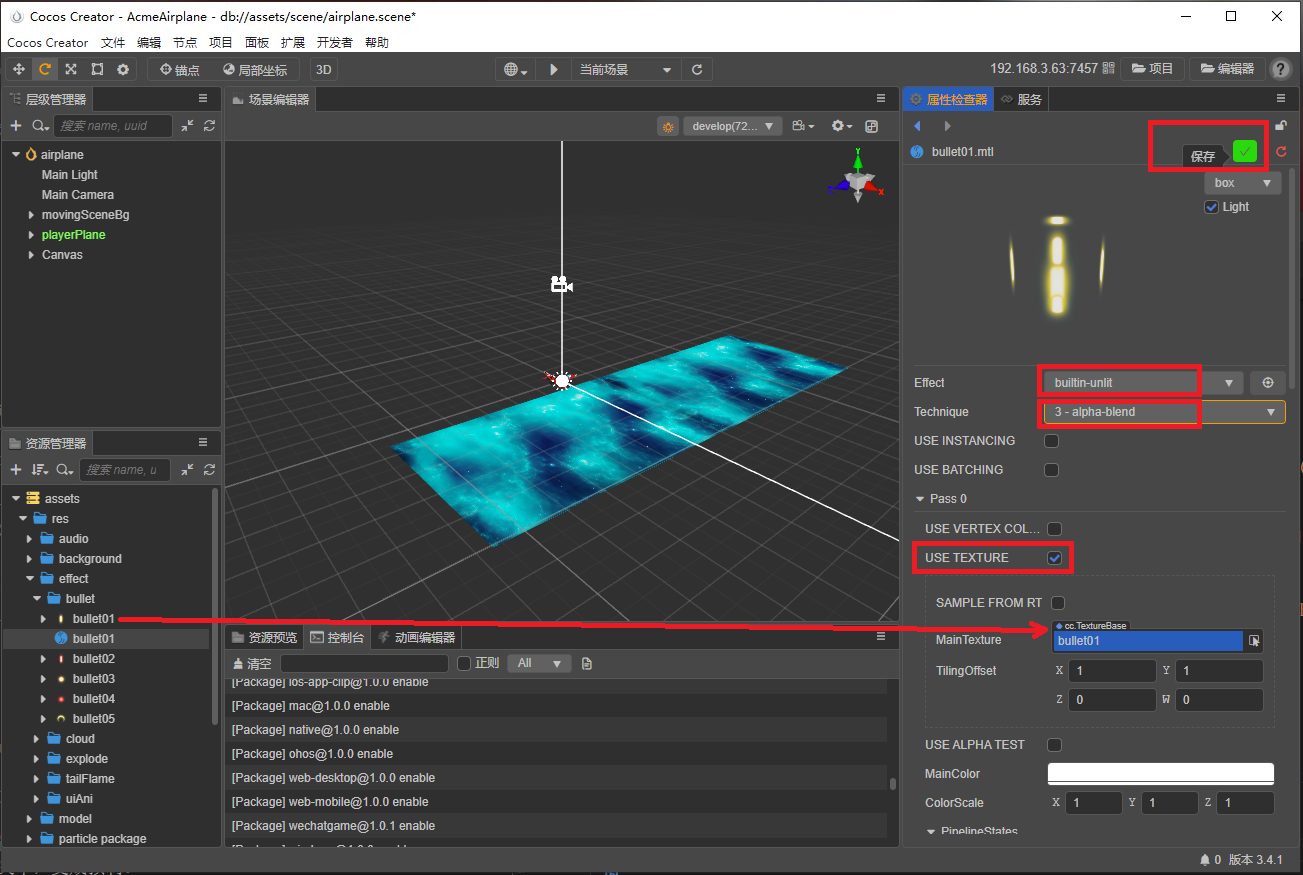
选中已经制作好的子弹材质
bullet01
,Ctrl+D
快速复制为4
个(bullet02
-bullet05
), 分别将各个新材质的属性MainTexture
更改为对应的图片, 保存。在
层级管理器
中创建一个名为bullet01
的空节点, 点击选中bullet01
节点,右键
->创建
->3D对象
->Quad(四方形)
, 将其改名为body
。创建好的
Quad(四方形)
垂直于背景, 将其Rotation
的X轴
改为-90
, 摆正其位置, 修改其Materials
为 材质bullet01
; 缩放属性Scale(缩放)
调整为5倍
大小,Scale: X:5, Y:5, Z:5
。
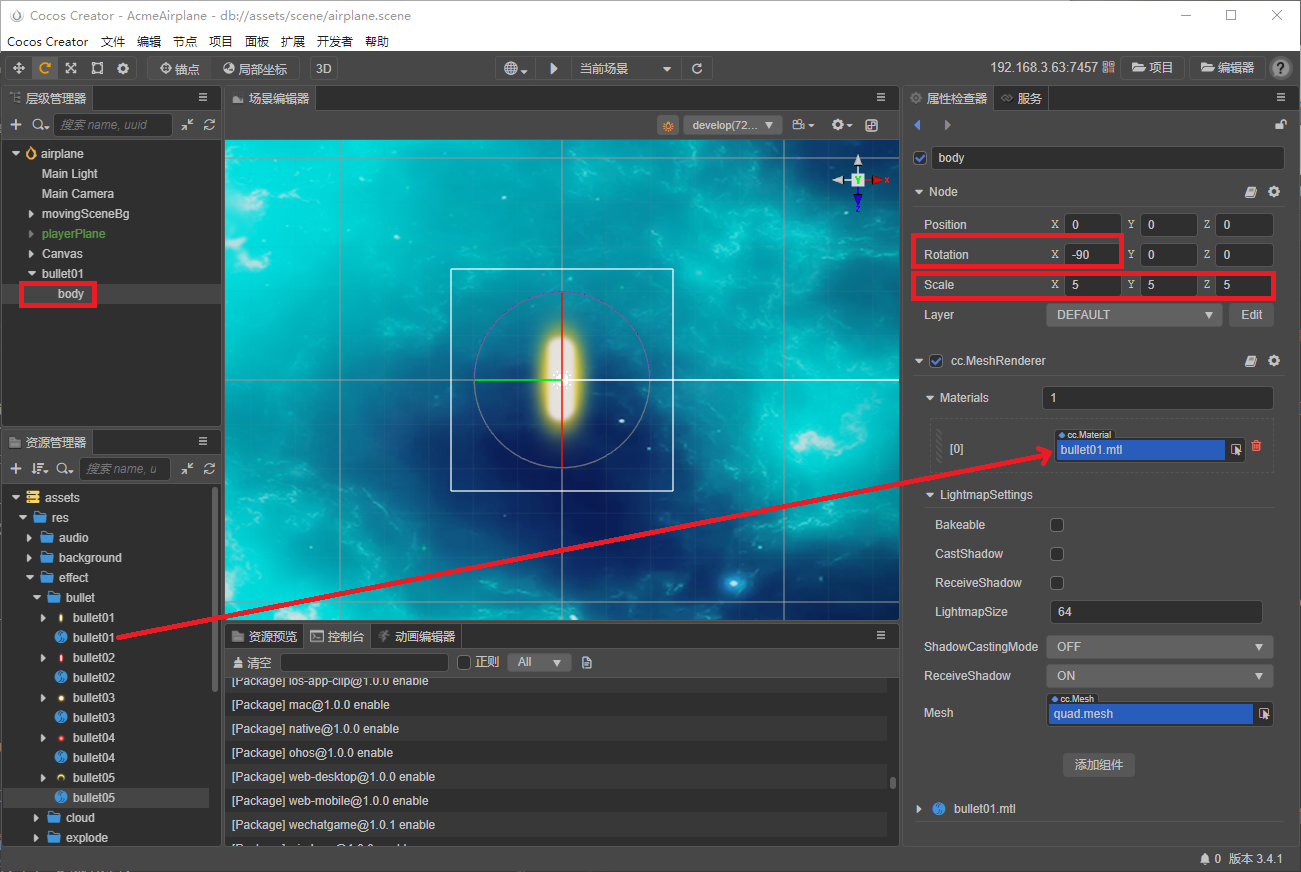
- 在
资源管理器
的script
文件夹中创建bullet
文件夹, 并创建子弹脚本Bullet
处理子弹的运行逻辑和子弹的销毁。
1 |
|
- 在
层级管理器
点击选中bullet01
节点, 在属性检查器
中点击添加组件
->自定义脚本
->Bullet
, 将脚本绑定到子弹根节点上; 然后将层级管理器
中bullet01
的节点拖动到资源管理器
的assets/res/effect/bullet/
文件夹中,变成预制。
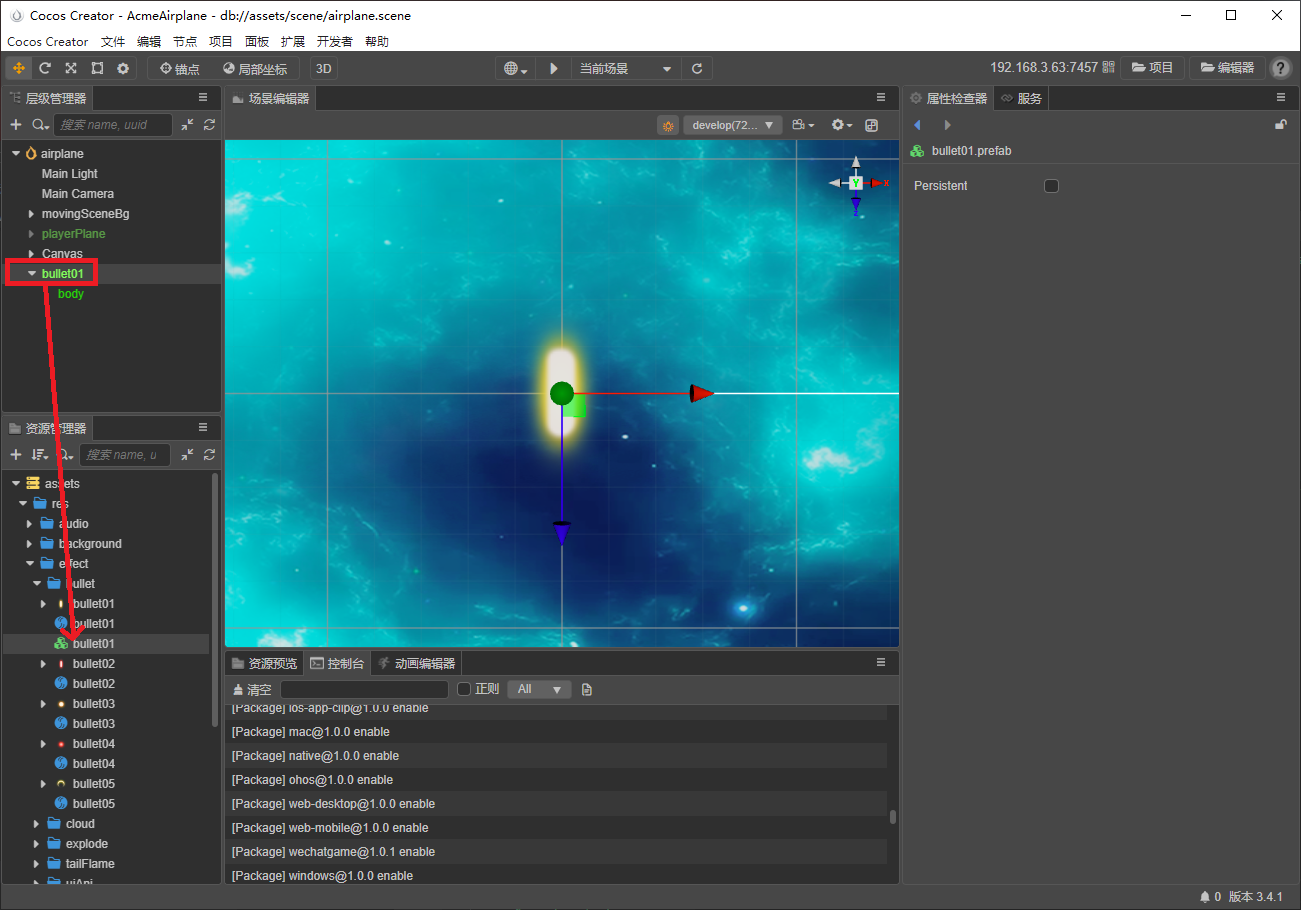
在
资源管理器
中点击选中assets/res/effect/bullet/bullet01
预制,Ctrl+D
快速复制为4
个(bullet02
-bullet05
)。在
资源管理器
中分别双击assets/res/effect/bullet/
文件夹中的预制bullet02
-bullet05
, 将body
中的Materials
替换为对应的子弹材质
, 保存场景。
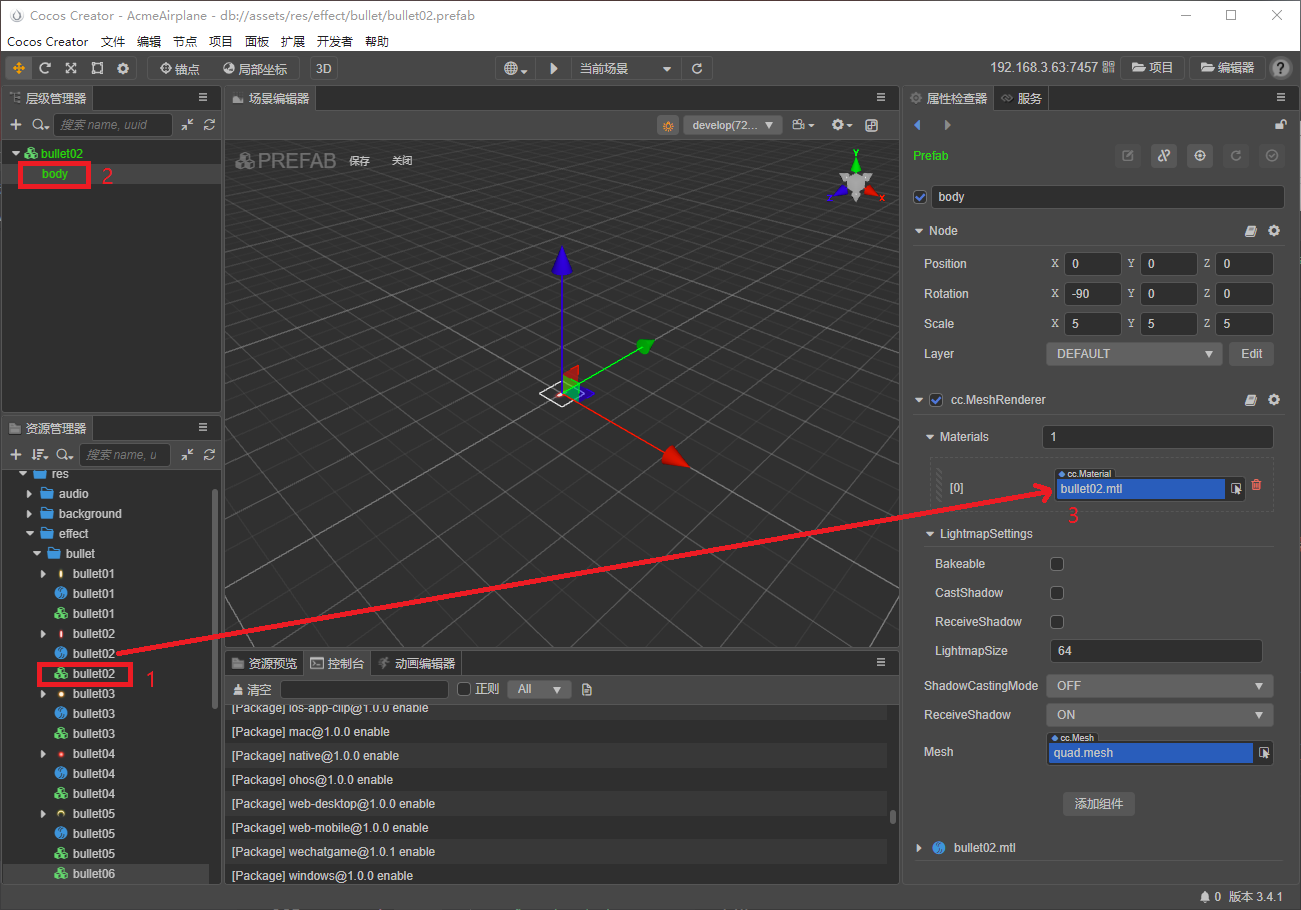
- 在
层级管理器
中删除
或不显示
(否则子弹会一直出现在屏幕上)子弹节点bullet01
, 并保存。
控制子弹发射
- 在
资源管理器
创建assets/script/framework
文件夹, 然后创建名为GameManager
的脚本。
1 |
|
- 修改
UIManager
脚本本以更配合GameManager
脚本使用
1 |
|
在
层级管理器
中点击选中场景airplane
,右键
->空节点
,命名为bulletManager
, 创建子弹管理节点。在
层级管理器
中点击选中场景airplane
,右键
->空节点
,命名为gameManager
, 在其属性检查器
中分别添加玩家飞机节点playerPlane
, 子弹预制:bullet01
-bullet05
, 子弹管理节点BulletRoot
:bulletManager
。
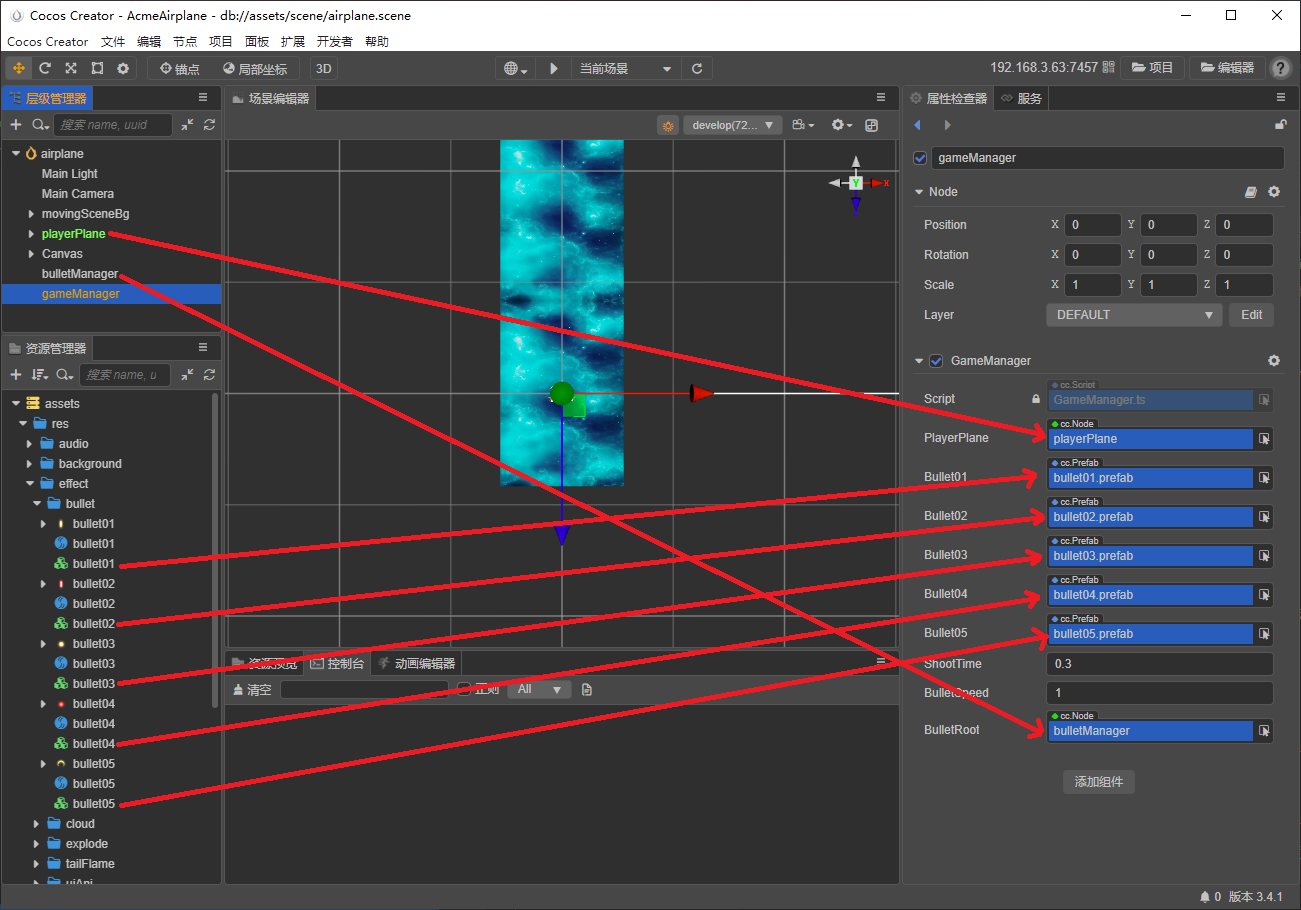
- 在
层级管理器
点击选中Canvas
节点, 将gameManager
节点拖动到其UIManamet
中GameManager
的属性。
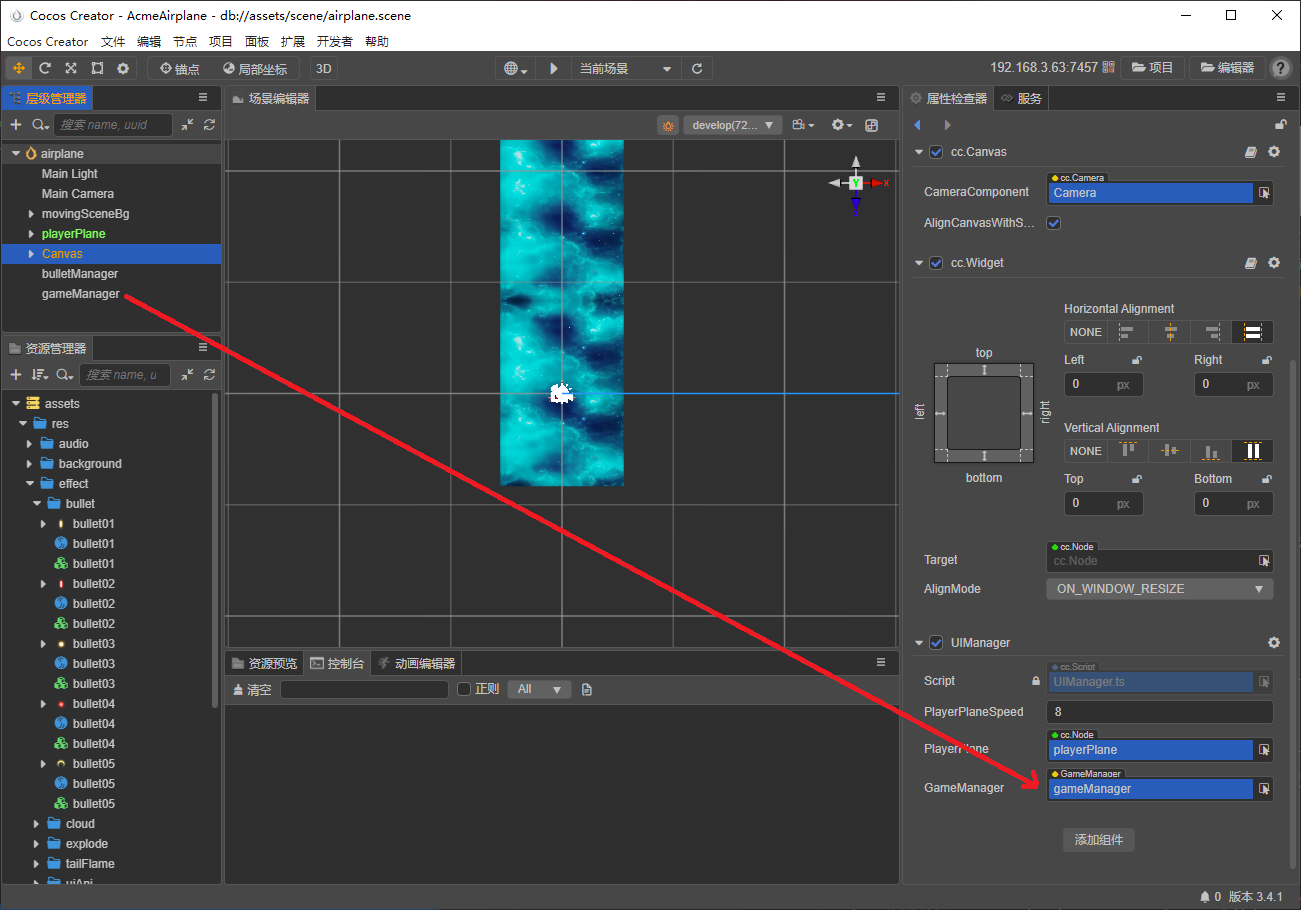
- 保存场景,预览。子弹速度慢,可以将
gameManager
节点 的shootTime
改为 0.1 , 缩短子弹发射间隔,提高速度,或是将bulletSpeed
值改大。
===END===